7.1.3. Multi- holes and slits.
In this example we demonstrate the use of a number of holes or slits in a row. In this way it is possible to make a grating. There are two wavelengths in the beam which demonstrates the use of a grating as a spectrometer. The intensities of the two fields can be simply added after separate propagations.
(Source code
, png
, hires.png
, pdf
)
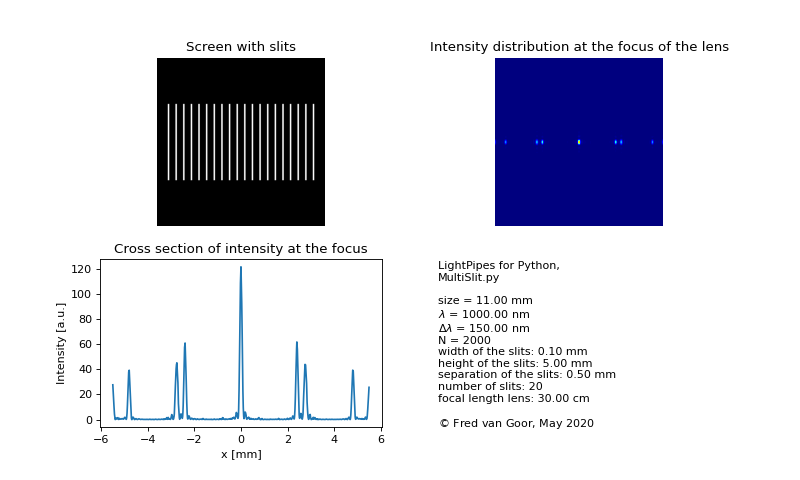
Below we demonstrate interference from a row of holes.
(Source code
, png
, hires.png
, pdf
)
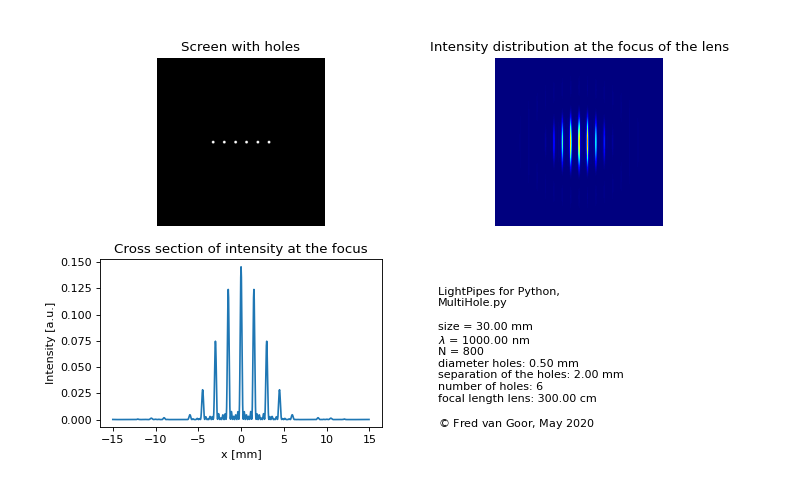